monaco-io/request
Fork: 28 Star: 291 (更新于 2024-11-13 05:59:36)
license: MIT
Language: Go .
go request, go http client
最后发布版本: v1.0.16 ( 2023-07-02 21:37:42)
Request

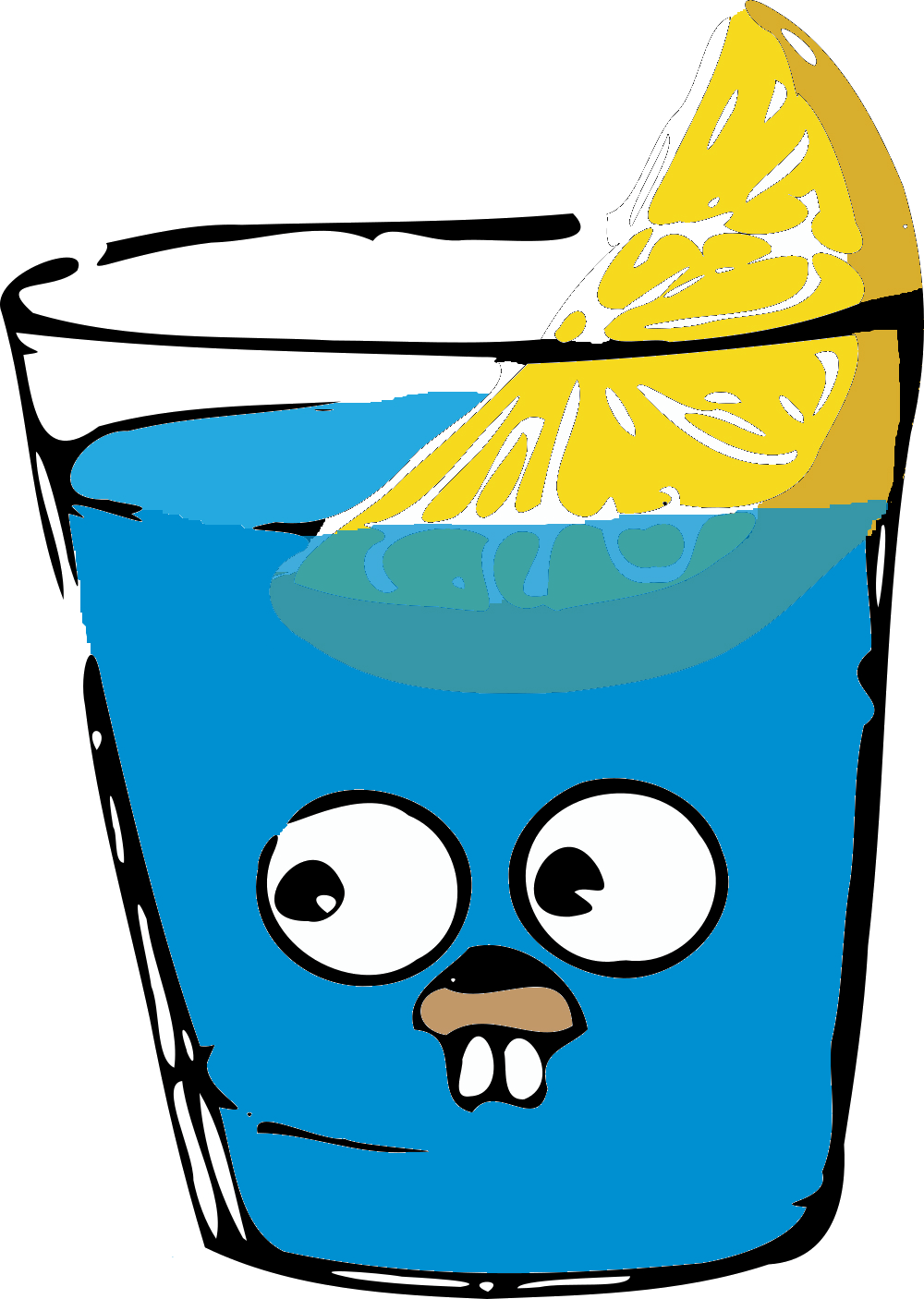
HTTP Client for golang, Inspired by Javascript-axios Python-request. If you have experience about axios or requests, you will love it. No 3rd dependency.
Features
- Make http requests from Golang
- Transform request and response data
Installing
go mod:
go get github.com/monaco-io/request
Methods
- OPTIONS
- GET
- HEAD
- POST
- PUT
- DELETE
- TRACE
- CONNECT
Example
POST
package main
import (
"github.com/monaco-io/request"
)
func main() {
var body = struct {
A string
B int
}{A: "A", B: 1}
var result interface{}
c := request.Client{
URL: "https://google.com",
Method: "POST",
Query: map[string]string{"hello": "world"},
JSON: body,
}
resp := c.Send().Scan(&result)
if !resp.OK(){
// handle error
log.Println(resp.Error())
}
// str := resp.String()
// bytes := resp.Bytes()
POST with local files
package main
import (
"github.com/monaco-io/request"
)
func main() {
c := request.Client{
URL: "https://google.com",
Method: "POST",
Query: map[string]string{"hello": "world"},
MultipartForm: MultipartForm{
Fields: map[string]string{"a": "1"},
Files: []string{"doc.txt"},
},
}
resp := c.Send().Scan(&result)
...
POST step by step
package main
import (
"github.com/monaco-io/request"
)
func main() {
var response interface{}
resp := request.
New().
POST("http://httpbin.org/post").
AddHeader(map[string]string{"Google": "google"}).
AddBasicAuth("google", "google").
AddURLEncodedForm(map[string]string{"data": "google"}).
Send().
Scan(&response)
...
POST with context (1/2)
package main
import (
"github.com/monaco-io/request"
"context"
)
func main() {
c := request.Client{
Context: context.Background(),
URL: "https://google.com",
Method: "POST",
BasicAuth: request.BasicAuth{
Username: "google",
Password: "google",
},
}
resp := c.Send()
...
POST with context (2/2)
package main
import (
"github.com/monaco-io/request"
"context"
)
func main() {
var response interface{}
resp := request.
NewWithContext(context.TODO()).
POST("http://httpbin.org/post").
AddHeader(map[string]string{"Google": "google"}).
AddBasicAuth("google", "google").
AddURLEncodedForm(map[string]string{"data": "google"}).
Send().
Scan(&response)
...
Authorization
package main
import (
"github.com/monaco-io/request"
)
func main() {
c := request.Client{
URL: "https://google.com",
Method: "POST",
BasicAuth: request.BasicAuth{
Username: "google",
Password: "google",
},
}
resp := c.Send()
}
Timeout
package main
import (
"github.com/monaco-io/request"
)
func main() {
c := request.Client{
URL: "https://google.com",
Method: "POST",
Timeout: time.Second*10,
}
}
Cookies
package main
import (
"github.com/monaco-io/request"
)
func main() {
c := request.Client{
URL: "https://google.com",
CookiesMap: map[string]string{
"cookie_name": "cookie_value",
}
}
}
TLS
package main
import (
"github.com/monaco-io/request"
)
func main() {
c := request.Client{
URL: "https://google.com",
TLSConfig: &tls.Config{InsecureSkipVerify: true},
}
}
License
最近版本更新:(数据更新于 2024-08-28 07:45:08)
2023-07-02 21:37:42 v1.0.16
2021-10-03 10:28:50 v1.0.15
2021-06-11 16:51:05 v1.0.14
2021-05-03 16:50:14 v1.0.13
2021-03-16 15:27:18 v1.0.12
2021-03-15 19:52:05 v1.0.11
2021-03-15 17:06:53 v1.0.10
2021-02-09 15:08:52 v1.0.9
2021-02-06 16:38:34 v1.0.8
2021-01-17 11:19:50 v1.0.7
主题(topics):
axios, client, go, golang, gorequest, http, net, request
monaco-io/request同语言 Go最近更新仓库
2024-11-21 22:49:20 containerd/containerd
2024-11-21 13:50:50 XTLS/Xray-core
2024-11-21 07:36:18 kubernetes/kubernetes
2024-11-21 06:27:30 ollama/ollama
2024-11-21 05:17:55 Melkeydev/go-blueprint
2024-11-21 04:04:03 dolthub/dolt