bun-v0.0.76
版本发布时间: 2022-04-08 16:33:35
oven-sh/bun最新发布版本:bun-v1.1.20(2024-07-13 14:04:45)
To upgrade:
bun upgrade
What's new
-
Type definitions for better editor integration and TypeScript LSP! There are types for the runtime APIs now in the
bun-types
npm package. A PR for @types/bun is waiting review (feel free to nudge them). -
Bun.serve()
is a fast HTTP & HTTPS server that supports theRequest
andResponse
Web APIs. The server is a fork of uWebSockets -
Bun.write()
– one API for writing files, pipes, and copying files leveraging the fastest system calls available for the input & platform. It uses theBlob
Web API. -
Bun.mmap(path)
lets you read files as a live-updatingUint8Array
via the mmap(2) syscall. Thank you @evanwashere!! -
Bun.hash(bufferOrString)
exposes fast non-cryptographic hashing functions. Useful for things likeETag
, not for passwords. -
Bun.allocUnsafe(length)
creates a new Uint8Array ~3.5x faster than new Uint8Array, but it is not zero-initialized. This is similar to Node'sBuffer.allocUnsafe
, though without the memory pool currently - Several web APIs have been added, including
URL
- A few more examples have been added to the
examples
folder - Bug fixes to
fs.read()
andfs.write()
and some more tests for fs -
Response.redirect()
,Response.json()
, andResponse.error()
have been added -
import.meta.url
is now a file:// url string -
Bun.resolve
andBun.resolveSync
let you resolve the same asimport
does. It throws aResolveError
on failure (same asimport
) -
Bun.stderr
andBun.stdout
now return aBlob
-
SharedArrayBuffer
is now enabled (thanks @evanwashere!) - Updated Next.js version
New Web APIs in bun.js
Going forward, Bun will first try to rely on WebKit/Safari's implementations of Web APIs rather than writing new ones. This will improve Web API compatibility while reducing bun's scope, without compromising performance
These Web APIs are now available in bun.js and powered by Safari's implementation:
-
URL
-
URLSearchParams
-
ErrorEvent
-
Event
-
EventTarget
-
DOMException
-
Headers
uses WebKit's implementation now instead of a custom one -
AbortSignal
(not wired up to fetch or fs yet, it exists but not very useful) -
AbortController
Also added:
-
reportError
(does not dispatch an"error"
event yet)
Additionally, all the builtin constructors in bun now have a .prototype
property (this was missing before)
Bun.serve
- fast HTTP server
For a hello world HTTP server that writes "bun!", Bun.serve
serves about 2.5x more requests per second than node.js on Linux:
Requests per second | Runtime |
---|---|
~64,000 | Node 16 |
~160,000 | Bun |
Bigger is better
Code
Bun:
Bun.serve({
fetch(req: Request) {
return new Response(`bun!`);
},
port: 3000,
});
Node:
require("http")
.createServer((req, res) => res.end("bun!"))
.listen(8080);
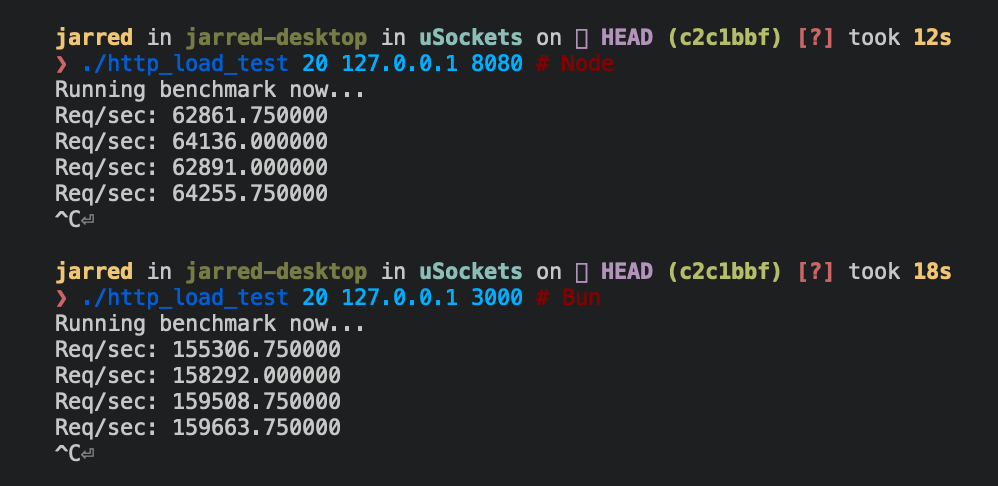
Usage
Two ways to start an HTTP server with bun.js:
-
export default
an object with afetch
function
If the file used to start bun has a default export with a fetch
function, it will start the http server.
// hi.js
export default {
fetch(req) {
return new Response("HI!");
},
};
// bun ./hi.js
fetch
receives a Request
object and must return either a Response
or a Promise<Response>
. In a future version, it might have an additional arguments for things like cookies.
-
Bun.serve
starts the http server explicitly
Bun.serve({
fetch(req) {
return new Response("HI!");
},
});
Error handling
For error handling, you get an error
function.
If development: true
and error
is not defined or doesn't return a Response
, you will get an exception page with a stack trace:
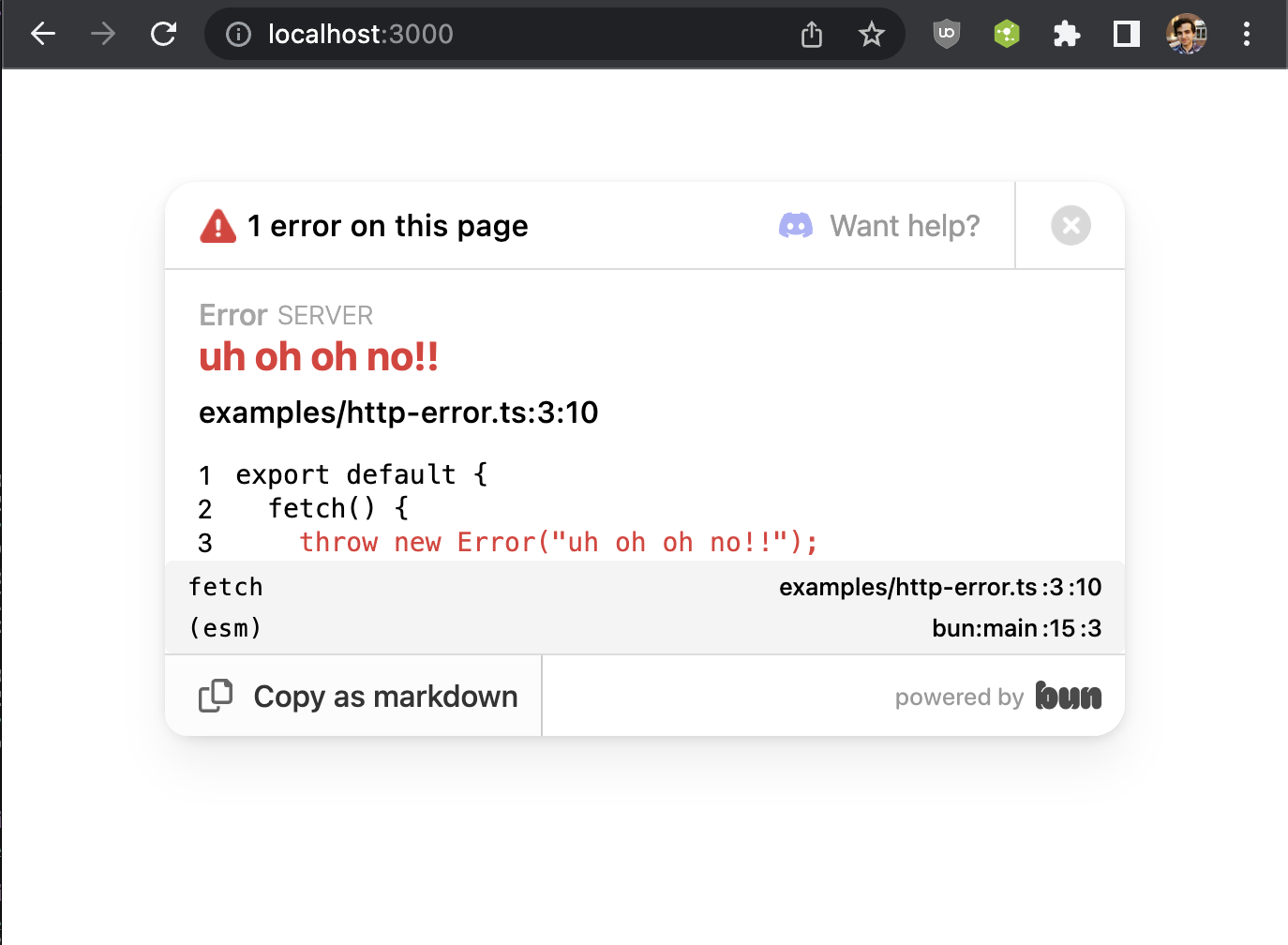
It will hopefully make it easier to debug issues with bun until bun gets debugger support. This error page is based on what bun dev
does.
If the error function returns a Response
, it will be served instead
Bun.serve({
fetch(req) {
throw new Error("woops!");
},
error(error: Error) {
return new Response("Uh oh!!\n" + error.toString(), { status: 500 });
},
});
If the error
function itself throws and development
is false
, a generic 500 page will be shown
Currently, there is no way to stop the HTTP server once started 😅, but that will be added in a future version.
The interface for Bun.serve
is based on what Cloudflare Workers does.
Bun.write() – optimizing I/O
Bun.write
lets you write, copy or pipe files automatically using the fastest system calls compatible with the input and platform.
interface Bun {
write(
destination: string | number | FileBlob,
input: string | FileBlob | Blob | ArrayBufferView
): Promise<number>;
}
Output | Input | System Call | Platform |
---|---|---|---|
file | file | copy_file_range | Linux |
file | pipe | sendfile | Linux |
pipe | pipe | splice | Linux |
terminal | file | sendfile | Linux |
terminal | terminal | sendfile | Linux |
socket | file or pipe | sendfile (if http, not https) | Linux |
file (path, doesn't exist) | file (path) | clonefile | macOS |
file | file | fcopyfile | macOS |
file | Blob or string | write | macOS |
file | Blob or string | write | Linux |
All this complexity is handled by a single function.
// Write "Hello World" to output.txt
await Bun.write("output.txt", "Hello World");
// log a file to stdout
await Bun.write(Bun.stdout, Bun.file("input.txt"));
// write the HTTP response body to disk
await Bun.write("index.html", await fetch("http://example.com"));
// this does the same thing
await Bun.write(Bun.file("index.html"), await fetch("http://example.com"));
// copy input.txt to output.txt
await Bun.write("output.txt", Bun.file("input.txt"));
Bug fixes
- Fixed a bug on Linux where sometimes the HTTP thread would incorrectly go to sleep, causing requests to hang forever 😢 . Previously, it relied on data in the queues to determine whether it should idle and now it increments a counter.
- Fixed a bug that sometimes caused
require
to produce incorrect output depending on how the module was used - Fixed a number of crashes in
bun dev
related to HMR & websockets - https://github.com/Jarred-Sumner/bun/commit/daeede28dbb3c6b5bb43250ca4f3524cd728ca4c -
fs.openSync
now supportsmode
andflags
- https://github.com/Jarred-Sumner/bun/commit/c73fcb073109405e1ccc30299bd9f8bef2791435 -
fs.read
andfs.write
were incorrectly returning the output offs/promises
versions, this is fixed - Fixed a crash that could occur during garbage collection when an
fs
function received a TypedArray as input. - https://github.com/Jarred-Sumner/bun/commit/614f64ba82947f7c2d3bf2dcc4e4993f6446e9b9. This also improves performance of sending array buffers to native a little -
Response
's constructor previously readstatusCode
instead ofstatus
. This was incorrect and has been fixed. - Fixed a bug where
fs.stat
reported incorrect information on macOS x64 - Slight improvement to HMR reliability if reading large files or slow filesystems over websockets - 89cd35f07fdb2e965e3518f3417e4c39f0163522
- Fixed a potential infinite loop when generating a sourcemap - fe973a5ab0d112ea6d80c8bcf7f693da879bb90d
improve performance of accessing
Bun.Transpiler
andBun.unsafe
29a759a65512278f1c20d1089ba05dbae268ef24
1、 bun-darwin-aarch64.dSYM.tar.gz 14.49MB
2、 bun-darwin-aarch64.zip 11.31MB
3、 bun-darwin-x64.dSYM.tar.gz 16.02MB
4、 bun-darwin-x64.zip 13.52MB
5、 bun-linux-x64.zip 26.61MB